mirror of
https://github.com/slawkens/myaac.git
synced 2025-07-02 01:30:14 +02:00
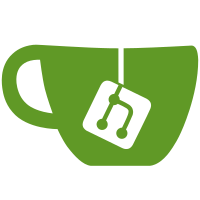
* Remove unneeded escape * Fix guild back buttons (change logo & motd) * small adjustment in news.php * Fix create character when admin (any case is allowed now) * Fix forum table style (boards & thread view) * Small improvement to plugins.enabled check * [WIP] nikic/fast-route implementation I will describe it more in Pull Request * Optimisations & fixes. * Fix path - should not be absolute * Add PLUGINS to Twig path * Don't hide "Install Plugin" Box by default * Update package-lock.json * nothing important, just early exit & fixes Fix creature display * fix premium_ends_at for tfs 1.3+ * Move pages * Move pages tbc * $db->select: make $where parameter optional, allows to get all records * Add some error box to error * fix parse error * Rewriting the router v2 To be more flexible * small fixes * fix & add admin icons * Move mass_* pages to correct folder * fix logout hook 2 * Delete accountmanagement.php * This code wasn't used * Add missing var * Add redirect_from && redirect_to to router options + Also add * for all methods shortcut * Remove comments Not allowed in normal json * Allow admin pages included into plugins dir * block access to some files * Fix admin logout * Fix #178 * feature: mail confirmed reward Suggested by @EPuncker # Conflicts: # system/hooks.php * remove misleading comment * adjust required version according to composer.json * fix duplicated word * Adjustments & fixed to mass actions * Add password confirm, and change text type to password * Add list of Open Source Software MyAAC is using * Fix signature * Show First, Second instead of numbers * fix base dir detection * fix double ACTION define + undefined URI in template * new function> escapeHtml + fix css in admin menus * fix changelog add * fix news adding, rename const to NEWS_* * Add verify to pages, add messages, limits, fix add * fix "Please fill all input" * add required input to admin pages * shorten some expressions with ?? * shorten code + fix conversion (int) * Move account_types to config, account.web_flags to common.php * Update example.json * feature: router aliases * shorten some code + const convert * remove wrong char * fix signature on custom basedir * fix: mass teleport position validation (#214) * fix: mass teleport position validation * fix: max position * Fix execute in CLI * fix warning in reload cache in dev mode * Configurable admin panel folder * feature: plugin require more options with comma * $config_account_salt -> USE_ACCOUNT_SALT * fix forum show_thread * Update show_thread.php --------- Co-authored-by: Gabriel Pedro <gpedro@users.noreply.github.com>
140 lines
5.2 KiB
PHP
140 lines
5.2 KiB
PHP
<?php
|
|
/**
|
|
* Menus
|
|
*
|
|
* @package MyAAC
|
|
* @author Slawkens <slawkens@gmail.com>
|
|
* @copyright 2019 MyAAC
|
|
* @link https://my-aac.org
|
|
*/
|
|
defined('MYAAC') or die('Direct access not allowed!');
|
|
$title = 'Menus';
|
|
|
|
if (!hasFlag(FLAG_CONTENT_MENUS) && !superAdmin()) {
|
|
echo 'Access denied.';
|
|
return;
|
|
}
|
|
|
|
if (isset($_REQUEST['template'])) {
|
|
$template = $_REQUEST['template'];
|
|
|
|
if (isset($_REQUEST['menu'])) {
|
|
$post_menu = $_REQUEST['menu'];
|
|
$post_menu_link = $_REQUEST['menu_link'];
|
|
$post_menu_blank = $_REQUEST['menu_blank'];
|
|
$post_menu_color = $_REQUEST['menu_color'];
|
|
if (count($post_menu) != count($post_menu_link)) {
|
|
echo 'Menu count is not equal menu links. Something went wrong when sending form.';
|
|
return;
|
|
}
|
|
|
|
$db->query('DELETE FROM `' . TABLE_PREFIX . 'menu` WHERE `template` = ' . $db->quote($template));
|
|
foreach ($post_menu as $category => $menus) {
|
|
foreach ($menus as $i => $menu) {
|
|
if (empty($menu)) // don't save empty menu item
|
|
continue;
|
|
|
|
try {
|
|
$db->insert(TABLE_PREFIX . 'menu', array('template' => $template, 'name' => $menu, 'link' => $post_menu_link[$category][$i], 'blank' => $post_menu_blank[$category][$i] == 'on' ? 1 : 0, 'color' => str_replace('#', '', $post_menu_color[$category][$i]), 'category' => $category, 'ordering' => $i));
|
|
} catch (PDOException $error) {
|
|
warning('Error while adding menu item (' . $menu . '): ' . $error->getMessage());
|
|
}
|
|
}
|
|
}
|
|
|
|
$cache = Cache::getInstance();
|
|
if ($cache->enabled()) {
|
|
$cache->delete('template_menus');
|
|
}
|
|
success('Saved at ' . date('H:i'));
|
|
}
|
|
|
|
$file = TEMPLATES . $template . '/config.php';
|
|
if (file_exists($file)) {
|
|
require_once $file;
|
|
} else {
|
|
echo 'Cannot find template config.php file.';
|
|
return;
|
|
}
|
|
if (!isset($config['menu_categories'])) {
|
|
echo "No menu categories set in template config.php.<br/>This template doesn't support dynamic menus.";
|
|
return;
|
|
}
|
|
|
|
$title = 'Menus - ' . $template;
|
|
?>
|
|
<div align="center" class="text-center">
|
|
<p class="note">You are editing: <?= $template ?><br/><br/>
|
|
Hint: You can drag menu items.<br/>
|
|
Hint: Add links to external sites using: <b>http://</b> or <b>https://</b> prefix.<br/>
|
|
Not all templates support blank and colorful links.
|
|
</p>
|
|
</div>
|
|
<?php
|
|
$menus = array();
|
|
$menus_db = $db->query('SELECT `name`, `link`, `blank`, `color`, `category`, `ordering` FROM `' . TABLE_PREFIX . 'menu` WHERE `enabled` = 1 AND `template` = ' . $db->quote($template) . ' ORDER BY `ordering` ASC;')->fetchAll();
|
|
foreach ($menus_db as $menu) {
|
|
$menus[$menu['category']][] = array('name' => $menu['name'], 'link' => $menu['link'], 'blank' => $menu['blank'], 'color' => $menu['color'], 'ordering' => $menu['ordering']);
|
|
}
|
|
$last_id = array();
|
|
?>
|
|
<form method="post" id="menus-form" action="?p=menus">
|
|
<input type="hidden" name="template" value="<?php echo $template ?>"/>
|
|
<div class="row">
|
|
<?php foreach ($config['menu_categories'] as $id => $cat): ?>
|
|
<div class="col-md-12 col-lg-6">
|
|
<div class="card card-info card-outline">
|
|
<div class="card-header">
|
|
<h5 class="m-0"><?php echo $cat['name'] ?> <i class="far fa-plus-square add-button" id="add-button-<?php echo $id ?>"></i></h5>
|
|
</div>
|
|
<div class="card-body">
|
|
<ul class="sortable" id="sortable-<?php echo $id ?>">
|
|
<?php
|
|
if (isset($menus[$id])) {
|
|
foreach ($menus[$id] as $i => $menu):
|
|
?>
|
|
<li class="ui-state-default" id="list-<?php echo $id ?>-<?php echo $i ?>"><label>Name:</label> <input type="text" name="menu[<?php echo $id ?>][]" value="<?php echo escapeHtml($menu['name']); ?>"/>
|
|
<label>Link:</label> <input type="text" name="menu_link[<?php echo $id ?>][]" value="<?php echo $menu['link'] ?>"/>
|
|
<input type="hidden" name="menu_blank[<?php echo $id ?>][]" value="0"/>
|
|
<label><input class="blank-checkbox" type="checkbox" <?php echo($menu['blank'] == 1 ? 'checked' : '') ?>/><span title="Open in New Window">New Window</span></label>
|
|
<input class="color-picker" type="text" name="menu_color[<?php echo $id ?>][]" value="#<?php echo $menu['color'] ?>"/>
|
|
<a class="remove-button" id="remove-button-<?php echo $id ?>-<?php echo $i ?>"><i class="fas fa-trash"></a></i></li>
|
|
<?php $last_id[$id] = $i;
|
|
endforeach;
|
|
} ?>
|
|
</ul>
|
|
</div>
|
|
</div>
|
|
</div>
|
|
<?php endforeach ?>
|
|
</div>
|
|
<div class="row pb-2">
|
|
<div class="col-md-12">
|
|
<button type="submit" class="btn btn-info"><i class="fas fa-update"></i> Save</button>
|
|
<?php
|
|
echo '<button type="button" class="btn btn-danger float-right" value="Cancel" onclick="window.location = \'' . ADMIN_URL . '?p=menus\';"><i class="fas fa-cancel"></i> Cancel</button>';
|
|
?>
|
|
</div>
|
|
</div>
|
|
</form>
|
|
<?php
|
|
$twig->display('admin.menus.js.html.twig', array(
|
|
'menus' => $menus,
|
|
'last_id' => $last_id
|
|
));
|
|
?>
|
|
<?php
|
|
} else {
|
|
$templates = $db->query('SELECT `template` FROM `' . TABLE_PREFIX . 'menu` GROUP BY `template`;')->fetchAll();
|
|
foreach ($templates as $key => $value) {
|
|
$file = TEMPLATES . $value['template'] . '/config.php';
|
|
if (!file_exists($file)) {
|
|
unset($templates[$key]);
|
|
}
|
|
}
|
|
|
|
$twig->display('admin.menus.form.html.twig', array(
|
|
'templates' => $templates
|
|
));
|
|
}
|