mirror of
https://github.com/slawkens/myaac.git
synced 2025-07-05 19:20:14 +02:00
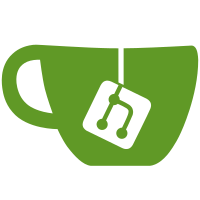
This fixes some errors on PHP 7.4 and contains even more fixes Also bumped PHP version to 5.5 as Twig requires it.
123 lines
3.5 KiB
PHP
123 lines
3.5 KiB
PHP
<?php
|
|
|
|
/*
|
|
* This file is part of Twig.
|
|
*
|
|
* (c) Fabien Potencier
|
|
* (c) Arnaud Le Blanc
|
|
*
|
|
* For the full copyright and license information, please view the LICENSE
|
|
* file that was distributed with this source code.
|
|
*/
|
|
|
|
use Twig\TokenParser\TokenParserInterface;
|
|
|
|
/**
|
|
* Default implementation of a token parser broker.
|
|
*
|
|
* @author Arnaud Le Blanc <arnaud.lb@gmail.com>
|
|
*
|
|
* @deprecated since 1.12 (to be removed in 2.0)
|
|
*/
|
|
class Twig_TokenParserBroker implements Twig_TokenParserBrokerInterface
|
|
{
|
|
protected $parser;
|
|
protected $parsers = [];
|
|
protected $brokers = [];
|
|
|
|
/**
|
|
* @param array|\Traversable $parsers A \Traversable of Twig_TokenParserInterface instances
|
|
* @param array|\Traversable $brokers A \Traversable of Twig_TokenParserBrokerInterface instances
|
|
* @param bool $triggerDeprecationError
|
|
*/
|
|
public function __construct($parsers = [], $brokers = [], $triggerDeprecationError = true)
|
|
{
|
|
if ($triggerDeprecationError) {
|
|
@trigger_error('The '.__CLASS__.' class is deprecated since version 1.12 and will be removed in 2.0.', E_USER_DEPRECATED);
|
|
}
|
|
|
|
foreach ($parsers as $parser) {
|
|
if (!$parser instanceof TokenParserInterface) {
|
|
throw new \LogicException('$parsers must a an array of Twig_TokenParserInterface.');
|
|
}
|
|
$this->parsers[$parser->getTag()] = $parser;
|
|
}
|
|
foreach ($brokers as $broker) {
|
|
if (!$broker instanceof Twig_TokenParserBrokerInterface) {
|
|
throw new \LogicException('$brokers must a an array of Twig_TokenParserBrokerInterface.');
|
|
}
|
|
$this->brokers[] = $broker;
|
|
}
|
|
}
|
|
|
|
public function addTokenParser(TokenParserInterface $parser)
|
|
{
|
|
$this->parsers[$parser->getTag()] = $parser;
|
|
}
|
|
|
|
public function removeTokenParser(TokenParserInterface $parser)
|
|
{
|
|
$name = $parser->getTag();
|
|
if (isset($this->parsers[$name]) && $parser === $this->parsers[$name]) {
|
|
unset($this->parsers[$name]);
|
|
}
|
|
}
|
|
|
|
public function addTokenParserBroker(self $broker)
|
|
{
|
|
$this->brokers[] = $broker;
|
|
}
|
|
|
|
public function removeTokenParserBroker(self $broker)
|
|
{
|
|
if (false !== $pos = array_search($broker, $this->brokers)) {
|
|
unset($this->brokers[$pos]);
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Gets a suitable TokenParser for a tag.
|
|
*
|
|
* First looks in parsers, then in brokers.
|
|
*
|
|
* @param string $tag A tag name
|
|
*
|
|
* @return TokenParserInterface|null A Twig_TokenParserInterface or null if no suitable TokenParser was found
|
|
*/
|
|
public function getTokenParser($tag)
|
|
{
|
|
if (isset($this->parsers[$tag])) {
|
|
return $this->parsers[$tag];
|
|
}
|
|
$broker = end($this->brokers);
|
|
while (false !== $broker) {
|
|
$parser = $broker->getTokenParser($tag);
|
|
if (null !== $parser) {
|
|
return $parser;
|
|
}
|
|
$broker = prev($this->brokers);
|
|
}
|
|
}
|
|
|
|
public function getParsers()
|
|
{
|
|
return $this->parsers;
|
|
}
|
|
|
|
public function getParser()
|
|
{
|
|
return $this->parser;
|
|
}
|
|
|
|
public function setParser(Twig_ParserInterface $parser)
|
|
{
|
|
$this->parser = $parser;
|
|
foreach ($this->parsers as $tokenParser) {
|
|
$tokenParser->setParser($parser);
|
|
}
|
|
foreach ($this->brokers as $broker) {
|
|
$broker->setParser($parser);
|
|
}
|
|
}
|
|
}
|