mirror of
https://github.com/slawkens/myaac.git
synced 2025-06-12 07:44:29 +02:00
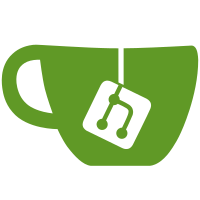
* New admin panel Pages: Options + Config [WIP] * Forgot the plugin example of options * Rename to settings.php * Add Settings Class * New myaac_settings table * Add $limit parameter to $db->select method * Add $member var annotation * Remove useless title_separator from config * Move $menus to menus.php Also fix active link when menu item has subpage * Settings [WIP] New Settings class New Plugins::load() method Move config.php to settings.php MyAAC Settings will have plugin_name = 'core' Add compat_config.php * Change options.php to settings.php * Change name to settings * Add Settings menu * Add Sections + Add setting($key) function Reorganisation * Add email + password fields as type * Update 33.php * add settings migration * php 8 compatibility * add missing hook * Add categories in tabs, move more settings, revert back getPluginSettings Categories and sections are now not numbered Remove example settings plugin * fix typo * Update .gitignore * Add 36th migration for settings table * Execute migrations just after db connect * Update plugins.php * [WIP] Some work on settings Add hidden settings New method: parse, to parse settings from array Move base html to twig template Remove vocation loading from .xml, instead use predefined voc names * Rename * Fix path * [WIP] More work on settings Move more config to settings (mainly mail_* + some other) Remove mail_admin, wasnt used anywhere Add return type to some functions Add Twig settings(key) function Possibility to save setting to db * Add min, max, step to number field option * Re-enable plugin if disabled and already installed * Add Settings menu, including all plugins with settings One change included in previous commit, due to missclick * Nothing important * Better boolean detection * More detailed error message in settings * Lets call it settings.name instead * Add new function: only_if, to hide fields when they are not enabled [WIP] Not fully finished yet * guild_management: show_if * Hide section title on show_if * Fix: check on page load if radio button is checked * Add: show_if - account_mail_verify * nothing important * Rename team_* variables + add to deprecated * Change variable name * Extract Settings:save function * Add settings.callbacks.get * Move forum config to settings * Move status config to settings * Remove whitespaces * More config to settings: account_types, genders, highscores, admin * Move signature config to settings * Move news config to settings * Rename variable * Save config.php in Settings Egg and hen problem solved :) * Test database connection on save settings -> prevents from making website unusable if connection is wrong * Test server_path -> same There is no config.php anymore, just config.local.php, which can be edited manually and also from admin panel * Remove configs from previous commit * Fix create account, if account_create_character_create is enabled * Add more deprecated configs * Add more info into comment * Update 5-database.php * Fix menu highlighting & opening * Update template.php * Enable script option * Reword email settings + move two new settings * add last_kills_limit + move shop * google_analytics_id * add mail_lost_account_interval * Create character blocked words (by @gpedro), just moved to settings * Fix google_analytics * create character name config moved to settings * Fix for install warning - min/max length * New create character checks configurable: block monsters & spells names * fixes * Improve character npc name check * New setting: donate_column + move donate config to settings * Add super fancy No Refresh saving with a toast * Add new possibility: to deny saving setting if condition is not met * Move database settings to separate category * Fix default value displaying * Add database_hash setting * add last_kills_limit to compat config * Move create character blocked names down * Every setting needs to have default * Move rest of config to settings Remove config.php completely Add new settings category: Game Fix account_login_by_email Min textarea size = 2 + adjusted automatically
151 lines
3.9 KiB
PHP
151 lines
3.9 KiB
PHP
<?php
|
|
/**
|
|
* Initialize some defaults
|
|
*
|
|
* @package MyAAC
|
|
* @author Slawkens <slawkens@gmail.com>
|
|
* @copyright 2019 MyAAC
|
|
* @link https://my-aac.org
|
|
*/
|
|
defined('MYAAC') or die('Direct access not allowed!');
|
|
|
|
if(!isset($config['installed']) || !$config['installed']) {
|
|
throw new RuntimeException('MyAAC has not been installed yet or there was error during installation. Please install again.');
|
|
}
|
|
|
|
if(config('env') === 'dev') {
|
|
require SYSTEM . 'exception.php';
|
|
}
|
|
|
|
if(empty($config['server_path'])) {
|
|
throw new RuntimeException('Server Path has been not set. Go to config.php and set it.');
|
|
}
|
|
|
|
// take care of trailing slash at the end
|
|
if($config['server_path'][strlen($config['server_path']) - 1] !== '/')
|
|
$config['server_path'] .= '/';
|
|
|
|
// enable gzip compression if supported by the browser
|
|
if(isset($config['gzip_output']) && $config['gzip_output'] && isset($_SERVER['HTTP_ACCEPT_ENCODING']) && strpos($_SERVER['HTTP_ACCEPT_ENCODING'], 'gzip') !== false && function_exists('ob_gzhandler'))
|
|
ob_start('ob_gzhandler');
|
|
|
|
// cache
|
|
require_once SYSTEM . 'libs/cache.php';
|
|
$cache = Cache::getInstance();
|
|
|
|
// twig
|
|
require_once SYSTEM . 'twig.php';
|
|
|
|
// action, used by many pages
|
|
$action = $_REQUEST['action'] ?? '';
|
|
define('ACTION', $action);
|
|
|
|
// trim values we receive
|
|
if(isset($_POST))
|
|
{
|
|
foreach($_POST as $var => $value) {
|
|
if(is_string($value)) {
|
|
$_POST[$var] = trim($value);
|
|
}
|
|
}
|
|
}
|
|
if(isset($_GET))
|
|
{
|
|
foreach($_GET as $var => $value) {
|
|
if(is_string($value))
|
|
$_GET[$var] = trim($value);
|
|
}
|
|
}
|
|
if(isset($_REQUEST))
|
|
{
|
|
foreach($_REQUEST as $var => $value) {
|
|
if(is_string($value))
|
|
$_REQUEST[$var] = trim($value);
|
|
}
|
|
}
|
|
|
|
// load otserv config file
|
|
$config_lua_reload = true;
|
|
if($cache->enabled()) {
|
|
$tmp = null;
|
|
if($cache->fetch('server_path', $tmp) && $tmp == $config['server_path']) {
|
|
$tmp = null;
|
|
if($cache->fetch('config_lua', $tmp) && $tmp) {
|
|
$config['lua'] = unserialize($tmp);
|
|
$config_lua_reload = false;
|
|
}
|
|
}
|
|
}
|
|
|
|
if($config_lua_reload) {
|
|
$config['lua'] = load_config_lua($config['server_path'] . 'config.lua');
|
|
|
|
// cache config
|
|
if($cache->enabled()) {
|
|
$cache->set('config_lua', serialize($config['lua']), 120);
|
|
$cache->set('server_path', $config['server_path']);
|
|
}
|
|
}
|
|
unset($tmp);
|
|
|
|
if(isset($config['lua']['servername']))
|
|
$config['lua']['serverName'] = $config['lua']['servername'];
|
|
|
|
if(isset($config['lua']['houserentperiod']))
|
|
$config['lua']['houseRentPeriod'] = $config['lua']['houserentperiod'];
|
|
|
|
// localize data/ directory based on data directory set in config.lua
|
|
foreach(array('dataDirectory', 'data_directory', 'datadir') as $key) {
|
|
if(!isset($config['lua'][$key][0])) {
|
|
break;
|
|
}
|
|
|
|
$foundValue = $config['lua'][$key];
|
|
if($foundValue[0] !== '/') {
|
|
$foundValue = $config['server_path'] . $foundValue;
|
|
}
|
|
|
|
if($foundValue[strlen($foundValue) - 1] !== '/') {// do not forget about trailing slash
|
|
$foundValue .= '/';
|
|
}
|
|
}
|
|
|
|
if(!isset($foundValue)) {
|
|
$foundValue = $config['server_path'] . 'data/';
|
|
}
|
|
|
|
$config['data_path'] = $foundValue;
|
|
unset($foundValue);
|
|
|
|
// POT
|
|
require_once SYSTEM . 'libs/pot/OTS.php';
|
|
$ots = POT::getInstance();
|
|
require_once SYSTEM . 'database.php';
|
|
|
|
// execute migrations
|
|
require SYSTEM . 'migrate.php';
|
|
|
|
// settings
|
|
require_once LIBS . 'Settings.php';
|
|
$settings = Settings::getInstance();
|
|
$settings->load();
|
|
|
|
// deprecated config values
|
|
require_once SYSTEM . 'compat/config.php';
|
|
|
|
date_default_timezone_set(setting('core.date_timezone'));
|
|
|
|
$config['account_create_character_create'] = config('account_create_character_create') && (!setting('core.mail_enabled') || !config('account_mail_verify'));
|
|
|
|
$settingsItemImagesURL = setting('core.item_images_url');
|
|
if($settingsItemImagesURL[strlen($settingsItemImagesURL) - 1] !== '/') {
|
|
setting(['core.item_images_url', $settingsItemImagesURL . '/']);
|
|
}
|
|
|
|
define('USE_ACCOUNT_NAME', $db->hasColumn('accounts', 'name'));
|
|
define('USE_ACCOUNT_NUMBER', $db->hasColumn('accounts', 'number'));
|
|
define('USE_ACCOUNT_SALT', $db->hasColumn('accounts', 'salt'));
|
|
|
|
require LIBS . 'Towns.php';
|
|
Towns::load();
|