mirror of
https://github.com/slawkens/myaac.git
synced 2025-04-26 09:19:22 +02:00
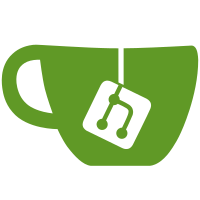
* New admin panel Pages: Options + Config [WIP] * Forgot the plugin example of options * Rename to settings.php * Add Settings Class * New myaac_settings table * Add $limit parameter to $db->select method * Add $member var annotation * Remove useless title_separator from config * Move $menus to menus.php Also fix active link when menu item has subpage * Settings [WIP] New Settings class New Plugins::load() method Move config.php to settings.php MyAAC Settings will have plugin_name = 'core' Add compat_config.php * Change options.php to settings.php * Change name to settings * Add Settings menu * Add Sections + Add setting($key) function Reorganisation * Add email + password fields as type * Update 33.php * add settings migration * php 8 compatibility * add missing hook * Add categories in tabs, move more settings, revert back getPluginSettings Categories and sections are now not numbered Remove example settings plugin * fix typo * Update .gitignore * Add 36th migration for settings table * Execute migrations just after db connect * Update plugins.php * [WIP] Some work on settings Add hidden settings New method: parse, to parse settings from array Move base html to twig template Remove vocation loading from .xml, instead use predefined voc names * Rename * Fix path * [WIP] More work on settings Move more config to settings (mainly mail_* + some other) Remove mail_admin, wasnt used anywhere Add return type to some functions Add Twig settings(key) function Possibility to save setting to db * Add min, max, step to number field option * Re-enable plugin if disabled and already installed * Add Settings menu, including all plugins with settings One change included in previous commit, due to missclick * Nothing important * Better boolean detection * More detailed error message in settings * Lets call it settings.name instead * Add new function: only_if, to hide fields when they are not enabled [WIP] Not fully finished yet * guild_management: show_if * Hide section title on show_if * Fix: check on page load if radio button is checked * Add: show_if - account_mail_verify * nothing important * Rename team_* variables + add to deprecated * Change variable name * Extract Settings:save function * Add settings.callbacks.get * Move forum config to settings * Move status config to settings * Remove whitespaces * More config to settings: account_types, genders, highscores, admin * Move signature config to settings * Move news config to settings * Rename variable * Save config.php in Settings Egg and hen problem solved :) * Test database connection on save settings -> prevents from making website unusable if connection is wrong * Test server_path -> same There is no config.php anymore, just config.local.php, which can be edited manually and also from admin panel * Remove configs from previous commit * Fix create account, if account_create_character_create is enabled * Add more deprecated configs * Add more info into comment * Update 5-database.php * Fix menu highlighting & opening * Update template.php * Enable script option * Reword email settings + move two new settings * add last_kills_limit + move shop * google_analytics_id * add mail_lost_account_interval * Create character blocked words (by @gpedro), just moved to settings * Fix google_analytics * create character name config moved to settings * Fix for install warning - min/max length * New create character checks configurable: block monsters & spells names * fixes * Improve character npc name check * New setting: donate_column + move donate config to settings * Add super fancy No Refresh saving with a toast * Add new possibility: to deny saving setting if condition is not met * Move database settings to separate category * Fix default value displaying * Add database_hash setting * add last_kills_limit to compat config * Move create character blocked names down * Every setting needs to have default * Move rest of config to settings Remove config.php completely Add new settings category: Game Fix account_login_by_email Min textarea size = 2 + adjusted automatically
137 lines
4.5 KiB
PHP
137 lines
4.5 KiB
PHP
<?php
|
|
/**
|
|
* Plugins
|
|
*
|
|
* @package MyAAC
|
|
* @author Slawkens <slawkens@gmail.com>
|
|
* @copyright 2019 MyAAC
|
|
* @link https://my-aac.org
|
|
*/
|
|
defined('MYAAC') or die('Direct access not allowed!');
|
|
$title = 'Plugin manager';
|
|
$use_datatable = true;
|
|
|
|
require_once LIBS . 'plugins.php';
|
|
|
|
if (!getBoolean(setting('core.admin_plugins_manage_enable'))) {
|
|
warning('Plugin installation and management is disabled in Settings.<br/>If you wish to enable, go to Settings and enable <strong>Enable Plugins Manage</strong>.');
|
|
}
|
|
else {
|
|
$twig->display('admin.plugins.form.html.twig');
|
|
|
|
if (isset($_REQUEST['uninstall'])) {
|
|
$uninstall = $_REQUEST['uninstall'];
|
|
|
|
if (Plugins::uninstall($uninstall)) {
|
|
success('Successfully uninstalled plugin ' . $uninstall);
|
|
} else {
|
|
error('Error while uninstalling plugin ' . $uninstall . ': ' . Plugins::getError());
|
|
}
|
|
} else if (isset($_REQUEST['enable'])) {
|
|
$enable = $_REQUEST['enable'];
|
|
if (Plugins::enable($enable)) {
|
|
success('Successfully enabled plugin ' . $enable);
|
|
} else {
|
|
error('Error while enabling plugin ' . $enable . ': ' . Plugins::getError());
|
|
}
|
|
} else if (isset($_REQUEST['disable'])) {
|
|
$disable = $_REQUEST['disable'];
|
|
if (Plugins::disable($disable)) {
|
|
success('Successfully disabled plugin ' . $disable);
|
|
} else {
|
|
error('Error while disabling plugin ' . $disable . ': ' . Plugins::getError());
|
|
}
|
|
} else if (isset($_FILES['plugin']['name'])) {
|
|
$file = $_FILES['plugin'];
|
|
$filename = $file['name'];
|
|
$tmp_name = $file['tmp_name'];
|
|
$type = $file['type'];
|
|
|
|
$name = explode('.', $filename);
|
|
$accepted_types = array('application/zip', 'application/x-zip-compressed', 'multipart/x-zip', 'application/x-compressed', 'application/octet-stream', 'application/zip-compressed');
|
|
|
|
if (isset($file['error'])) {
|
|
$error = 'Error uploading file';
|
|
switch ($file['error']) {
|
|
case UPLOAD_ERR_OK:
|
|
$error = false;
|
|
break;
|
|
case UPLOAD_ERR_INI_SIZE:
|
|
case UPLOAD_ERR_FORM_SIZE:
|
|
$error .= ' - file too large (limit of ' . ini_get('upload_max_filesize') . ' bytes). You can enlarge the limits by changing "upload_max_filesize" in php.ini';
|
|
break;
|
|
case UPLOAD_ERR_PARTIAL:
|
|
$error .= ' - file upload was not completed.';
|
|
break;
|
|
case UPLOAD_ERR_NO_FILE:
|
|
$error .= ' - zero-length file uploaded.';
|
|
break;
|
|
default:
|
|
$error .= ' - internal error #' . $file['error'];
|
|
break;
|
|
}
|
|
}
|
|
|
|
if (isset($error) && $error != false) {
|
|
error($error);
|
|
} else {
|
|
if (is_uploaded_file($file['tmp_name'])) {
|
|
$filetype = strtolower(pathinfo($filename, PATHINFO_EXTENSION));
|
|
if ($filetype == 'zip') // check if it is zipped/compressed file
|
|
{
|
|
$tmp_filename = pathinfo($filename, PATHINFO_FILENAME);
|
|
$targetzip = BASE . 'plugins/' . $tmp_filename . '.zip';
|
|
|
|
if (move_uploaded_file($tmp_name, $targetzip)) { // move uploaded file
|
|
if (Plugins::install($targetzip)) {
|
|
foreach (Plugins::getWarnings() as $warning) {
|
|
warning($warning);
|
|
}
|
|
|
|
$info = Plugins::getPluginJson();
|
|
success((isset($info['name']) ? '<strong>' . $info['name'] . '</strong> p' : 'P') . 'lugin has been successfully installed.');
|
|
} else {
|
|
$error = Plugins::getError();
|
|
error(!empty($error) ? $error : 'Unexpected error happened while installing plugin. Please try again later.');
|
|
}
|
|
|
|
unlink($targetzip); // delete the Zipped file
|
|
} else
|
|
error('There was a problem with the upload. Please try again.');
|
|
} else {
|
|
error('The file you are trying to upload is not a .zip file. Please try again.');
|
|
}
|
|
} else {
|
|
error('Error uploading file - unknown error.');
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
$plugins = array();
|
|
foreach (get_plugins(true) as $plugin) {
|
|
$string = file_get_contents(BASE . 'plugins/' . $plugin . '.json');
|
|
$plugin_info = json_decode($string, true);
|
|
|
|
if (!$plugin_info) {
|
|
warning('Cannot load plugin info ' . $plugin . '.json');
|
|
} else {
|
|
$disabled = (strpos($plugin, 'disabled.') !== false);
|
|
$pluginOriginal = ($disabled ? str_replace('disabled.', '', $plugin) : $plugin);
|
|
$plugins[] = array(
|
|
'name' => $plugin_info['name'] ?? '',
|
|
'description' => $plugin_info['description'] ?? '',
|
|
'version' => $plugin_info['version'] ?? '',
|
|
'author' => $plugin_info['author'] ?? '',
|
|
'contact' => $plugin_info['contact'] ?? '',
|
|
'file' => $pluginOriginal,
|
|
'enabled' => !$disabled,
|
|
'uninstall' => isset($plugin_info['uninstall'])
|
|
);
|
|
}
|
|
}
|
|
|
|
$twig->display('admin.plugins.html.twig', array(
|
|
'plugins' => $plugins
|
|
));
|