mirror of
https://github.com/slawkens/myaac.git
synced 2025-07-02 01:30:14 +02:00
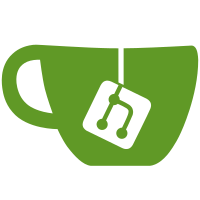
This fixes some errors on PHP 7.4 and contains even more fixes Also bumped PHP version to 5.5 as Twig requires it.
90 lines
2.0 KiB
PHP
Executable File
90 lines
2.0 KiB
PHP
Executable File
<?php
|
|
|
|
/*
|
|
* This file is part of Twig.
|
|
*
|
|
* (c) Fabien Potencier
|
|
*
|
|
* For the full copyright and license information, please view the LICENSE
|
|
* file that was distributed with this source code.
|
|
*/
|
|
|
|
namespace Twig;
|
|
|
|
use Twig\NodeVisitor\NodeVisitorInterface;
|
|
|
|
/**
|
|
* A node traverser.
|
|
*
|
|
* It visits all nodes and their children and calls the given visitor for each.
|
|
*
|
|
* @final
|
|
*
|
|
* @author Fabien Potencier <fabien@symfony.com>
|
|
*/
|
|
class NodeTraverser
|
|
{
|
|
protected $env;
|
|
protected $visitors = [];
|
|
|
|
/**
|
|
* @param NodeVisitorInterface[] $visitors
|
|
*/
|
|
public function __construct(Environment $env, array $visitors = [])
|
|
{
|
|
$this->env = $env;
|
|
foreach ($visitors as $visitor) {
|
|
$this->addVisitor($visitor);
|
|
}
|
|
}
|
|
|
|
public function addVisitor(NodeVisitorInterface $visitor)
|
|
{
|
|
$this->visitors[$visitor->getPriority()][] = $visitor;
|
|
}
|
|
|
|
/**
|
|
* Traverses a node and calls the registered visitors.
|
|
*
|
|
* @return \Twig_NodeInterface
|
|
*/
|
|
public function traverse(\Twig_NodeInterface $node)
|
|
{
|
|
ksort($this->visitors);
|
|
foreach ($this->visitors as $visitors) {
|
|
foreach ($visitors as $visitor) {
|
|
$node = $this->traverseForVisitor($visitor, $node);
|
|
}
|
|
}
|
|
|
|
return $node;
|
|
}
|
|
|
|
protected function traverseForVisitor(NodeVisitorInterface $visitor, \Twig_NodeInterface $node = null)
|
|
{
|
|
if (null === $node) {
|
|
return;
|
|
}
|
|
|
|
$node = $visitor->enterNode($node, $this->env);
|
|
|
|
foreach ($node as $k => $n) {
|
|
if (null === $n) {
|
|
continue;
|
|
}
|
|
|
|
if (false !== ($m = $this->traverseForVisitor($visitor, $n)) && null !== $m) {
|
|
if ($m !== $n) {
|
|
$node->setNode($k, $m);
|
|
}
|
|
} else {
|
|
$node->removeNode($k);
|
|
}
|
|
}
|
|
|
|
return $visitor->leaveNode($node, $this->env);
|
|
}
|
|
}
|
|
|
|
class_alias('Twig\NodeTraverser', 'Twig_NodeTraverser');
|