mirror of
https://github.com/slawkens/myaac.git
synced 2025-07-05 19:20:14 +02:00
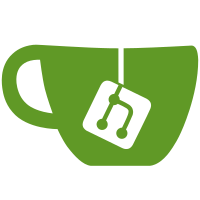
* this requires loaded items - go to admin panel and select Items menu, then reload * added items.xml loader class and weapons.xml loader class * load also runes into spells table * (internal) changed spells.vocations database field to store json data instead of comma separated * (internal) renamed existing Items class to Items_Images
192 lines
5.4 KiB
PHP
192 lines
5.4 KiB
PHP
<?php
|
|
/**
|
|
* Spells class
|
|
*
|
|
* @package MyAAC
|
|
* @author Gesior <jerzyskalski@wp.pl>
|
|
* @author Slawkens <slawkens@gmail.com>
|
|
* @copyright 2017 MyAAC
|
|
* @version 0.6.1
|
|
* @link http://my-aac.org
|
|
*/
|
|
defined('MYAAC') or die('Direct access not allowed!');
|
|
|
|
class Spells {
|
|
private static $spellsList = null;
|
|
private static $lastError = '';
|
|
|
|
public static function loadFromXML($show = false) {
|
|
global $config, $db;
|
|
|
|
try { $db->query('DELETE FROM `' . TABLE_PREFIX . 'spells`;'); } catch(PDOException $error) {}
|
|
|
|
if($show) {
|
|
echo '<h2>Reload spells.</h2>';
|
|
echo '<h2>All records deleted from table <b>' . TABLE_PREFIX . 'spells</b> in database.</h2>';
|
|
}
|
|
|
|
foreach($config['vocations'] as $voc_id => $voc_name) {
|
|
$vocations_ids[$voc_name] = $voc_id;
|
|
}
|
|
|
|
try {
|
|
self::$spellsList = new OTS_SpellsList($config['data_path'].'spells/spells.xml');
|
|
}
|
|
catch(Exception $e) {
|
|
self::$lastError = $e->getMessage();
|
|
return false;
|
|
}
|
|
//add conjure spells
|
|
$conjurelist = self::$spellsList->getConjuresList();
|
|
if($show) {
|
|
echo "<h3>Conjure:</h3>";
|
|
}
|
|
|
|
foreach($conjurelist as $spellname) {
|
|
$spell = self::$spellsList->getConjure($spellname);
|
|
$lvl = $spell->getLevel();
|
|
$mlvl = $spell->getMagicLevel();
|
|
$mana = $spell->getMana();
|
|
$name = $spell->getName();
|
|
$soul = $spell->getSoul();
|
|
$spell_txt = $spell->getWords();
|
|
|
|
$vocations = $spell->getVocations();
|
|
|
|
$enabled = $spell->isEnabled();
|
|
if($enabled) {
|
|
$hide_spell = 0;
|
|
}
|
|
else {
|
|
$hide_spell = 1;
|
|
}
|
|
$pacc = $spell->isPremium();
|
|
if($pacc) {
|
|
$pacc = '1';
|
|
}
|
|
else {
|
|
$pacc = '0';
|
|
}
|
|
$type = 2;
|
|
$count = $spell->getConjureCount();
|
|
try {
|
|
$db->query('INSERT INTO myaac_spells (spell, name, words, type, mana, level, maglevel, soul, premium, vocations, conjure_count, hidden) VALUES (' . $db->quote($spell_txt) . ', ' . $db->quote($name) . ', ' . $db->quote($spell_txt) . ', ' . $db->quote($type) . ', ' . $db->quote($mana) . ', ' . $db->quote($lvl) . ', ' . $db->quote($mlvl) . ', ' . $db->quote($soul) . ', ' . $db->quote($pacc) . ', ' . $db->quote(json_encode($vocations)) . ', ' . $db->quote($count) . ', ' . $db->quote($hide_spell) . ')');
|
|
if($show) {
|
|
success("Added: " . $name . "<br>");
|
|
}
|
|
}
|
|
catch(PDOException $error) {
|
|
if($show) {
|
|
warning('Error while adding spell (' . $name . '): ' . $error->getMessage());
|
|
}
|
|
}
|
|
}
|
|
|
|
//add instant spells
|
|
$instantlist = self::$spellsList->getInstantsList();
|
|
if($show) {
|
|
echo "<h3>Instant:</h3>";
|
|
}
|
|
|
|
foreach($instantlist as $spellname) {
|
|
$spell = self::$spellsList->getInstant($spellname);
|
|
$lvl = $spell->getLevel();
|
|
$mlvl = $spell->getMagicLevel();
|
|
$mana = $spell->getMana();
|
|
$name = $spell->getName();
|
|
$soul = $spell->getSoul();
|
|
$spell_txt = $spell->getWords();
|
|
if(strpos($spell_txt, '###') !== false)
|
|
continue;
|
|
|
|
$vocations = $spell->getVocations();
|
|
|
|
$enabled = $spell->isEnabled();
|
|
if($enabled) {
|
|
$hide_spell = 0;
|
|
}
|
|
else {
|
|
$hide_spell = 1;
|
|
}
|
|
$pacc = $spell->isPremium();
|
|
if($pacc) {
|
|
$pacc = '1';
|
|
}
|
|
else {
|
|
$pacc = '0';
|
|
}
|
|
$type = 1;
|
|
$count = 0;
|
|
|
|
|
|
try {
|
|
$db->query("INSERT INTO myaac_spells (spell, name, words, type, mana, level, maglevel, soul, premium, vocations, conjure_count, hidden) VALUES (".$db->quote($spell_txt).", ".$db->quote($name).", ".$db->quote($spell_txt).", '".$type."', '".$mana."', '".$lvl."', '".$mlvl."', '".$soul."', '".$pacc."', ".$db->quote(json_encode($vocations)).", '".$count."', '".$hide_spell."')");
|
|
if($show) {
|
|
success("Added: ".$name."<br/>");
|
|
}
|
|
}
|
|
catch(PDOException $error) {
|
|
if($show) {
|
|
warning('Error while adding spell (' . $name . '): ' . $error->getMessage());
|
|
}
|
|
}
|
|
}
|
|
|
|
//add runes
|
|
$runeslist = self::$spellsList->getRunesList();
|
|
if($show) {
|
|
echo "<h3>Runes:</h3>";
|
|
}
|
|
// runes
|
|
foreach($runeslist as $spellname) {
|
|
$spell = self::$spellsList->getRune($spellname);
|
|
$lvl = $spell->getLevel();
|
|
$mlvl = $spell->getMagicLevel();
|
|
$mana = $spell->getMana();
|
|
$name = $spell->getName() . ' (rune)';
|
|
$soul = $spell->getSoul();
|
|
$spell_txt = $spell->getWords();
|
|
$vocations = $spell->getVocations();
|
|
|
|
$id = $spell->getID();
|
|
|
|
$enabled = $spell->isEnabled();
|
|
if($enabled) {
|
|
$hide_spell = 0;
|
|
}
|
|
else {
|
|
$hide_spell = 1;
|
|
}
|
|
$pacc = $spell->isPremium();
|
|
if($pacc) {
|
|
$pacc = '1';
|
|
}
|
|
else {
|
|
$pacc = '0';
|
|
}
|
|
$type = 3;
|
|
$count = $spell->getConjureCount();
|
|
try {
|
|
$db->query('INSERT INTO myaac_spells (spell, name, words, type, mana, level, maglevel, soul, premium, vocations, conjure_count, item_id, hidden) VALUES (' . $db->quote($spell_txt) . ', ' . $db->quote($name) . ', ' . $db->quote($spell_txt) . ', ' . $db->quote($type) . ', ' . $db->quote($mana) . ', ' . $db->quote($lvl) . ', ' . $db->quote($mlvl) . ', ' . $db->quote($soul) . ', ' . $db->quote($pacc) . ', ' . $db->quote(json_encode($vocations)) . ', ' . $db->quote($count) . ', ' . $db->quote($id) . ', ' . $db->quote($hide_spell) . ')');
|
|
if($show) {
|
|
success("Added: " . $name . "<br>");
|
|
}
|
|
}
|
|
catch(PDOException $error) {
|
|
if($show) {
|
|
warning('Error while adding spell (' . $name . '): ' . $error->getMessage());
|
|
}
|
|
}
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
public static function getSpellsList() {
|
|
return self::$spellsList;
|
|
}
|
|
|
|
public static function getLastError() {
|
|
return self::$lastError;
|
|
}
|
|
} |