mirror of
https://github.com/slawkens/myaac.git
synced 2025-07-10 22:08:32 +02:00
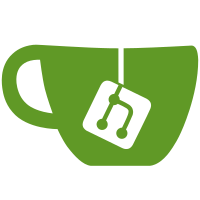
This fixes some errors on PHP 7.4 and contains even more fixes Also bumped PHP version to 5.5 as Twig requires it.
165 lines
4.5 KiB
PHP
165 lines
4.5 KiB
PHP
<?php
|
|
|
|
/*
|
|
* This file is part of Twig.
|
|
*
|
|
* (c) Fabien Potencier
|
|
*
|
|
* For the full copyright and license information, please view the LICENSE
|
|
* file that was distributed with this source code.
|
|
*/
|
|
|
|
namespace Twig\Loader;
|
|
|
|
use Twig\Error\LoaderError;
|
|
use Twig\Source;
|
|
|
|
/**
|
|
* Loads templates from other loaders.
|
|
*
|
|
* @final
|
|
*
|
|
* @author Fabien Potencier <fabien@symfony.com>
|
|
*/
|
|
class ChainLoader implements LoaderInterface, ExistsLoaderInterface, SourceContextLoaderInterface
|
|
{
|
|
private $hasSourceCache = [];
|
|
protected $loaders = [];
|
|
|
|
/**
|
|
* @param LoaderInterface[] $loaders
|
|
*/
|
|
public function __construct(array $loaders = [])
|
|
{
|
|
foreach ($loaders as $loader) {
|
|
$this->addLoader($loader);
|
|
}
|
|
}
|
|
|
|
public function addLoader(LoaderInterface $loader)
|
|
{
|
|
$this->loaders[] = $loader;
|
|
$this->hasSourceCache = [];
|
|
}
|
|
|
|
/**
|
|
* @return LoaderInterface[]
|
|
*/
|
|
public function getLoaders()
|
|
{
|
|
return $this->loaders;
|
|
}
|
|
|
|
public function getSource($name)
|
|
{
|
|
@trigger_error(sprintf('Calling "getSource" on "%s" is deprecated since 1.27. Use getSourceContext() instead.', \get_class($this)), E_USER_DEPRECATED);
|
|
|
|
$exceptions = [];
|
|
foreach ($this->loaders as $loader) {
|
|
if ($loader instanceof ExistsLoaderInterface && !$loader->exists($name)) {
|
|
continue;
|
|
}
|
|
|
|
try {
|
|
return $loader->getSource($name);
|
|
} catch (LoaderError $e) {
|
|
$exceptions[] = $e->getMessage();
|
|
}
|
|
}
|
|
|
|
throw new LoaderError(sprintf('Template "%s" is not defined%s.', $name, $exceptions ? ' ('.implode(', ', $exceptions).')' : ''));
|
|
}
|
|
|
|
public function getSourceContext($name)
|
|
{
|
|
$exceptions = [];
|
|
foreach ($this->loaders as $loader) {
|
|
if ($loader instanceof ExistsLoaderInterface && !$loader->exists($name)) {
|
|
continue;
|
|
}
|
|
|
|
try {
|
|
if ($loader instanceof SourceContextLoaderInterface) {
|
|
return $loader->getSourceContext($name);
|
|
}
|
|
|
|
return new Source($loader->getSource($name), $name);
|
|
} catch (LoaderError $e) {
|
|
$exceptions[] = $e->getMessage();
|
|
}
|
|
}
|
|
|
|
throw new LoaderError(sprintf('Template "%s" is not defined%s.', $name, $exceptions ? ' ('.implode(', ', $exceptions).')' : ''));
|
|
}
|
|
|
|
public function exists($name)
|
|
{
|
|
$name = (string) $name;
|
|
|
|
if (isset($this->hasSourceCache[$name])) {
|
|
return $this->hasSourceCache[$name];
|
|
}
|
|
|
|
foreach ($this->loaders as $loader) {
|
|
if ($loader instanceof ExistsLoaderInterface) {
|
|
if ($loader->exists($name)) {
|
|
return $this->hasSourceCache[$name] = true;
|
|
}
|
|
|
|
continue;
|
|
}
|
|
|
|
try {
|
|
if ($loader instanceof SourceContextLoaderInterface) {
|
|
$loader->getSourceContext($name);
|
|
} else {
|
|
$loader->getSource($name);
|
|
}
|
|
|
|
return $this->hasSourceCache[$name] = true;
|
|
} catch (LoaderError $e) {
|
|
}
|
|
}
|
|
|
|
return $this->hasSourceCache[$name] = false;
|
|
}
|
|
|
|
public function getCacheKey($name)
|
|
{
|
|
$exceptions = [];
|
|
foreach ($this->loaders as $loader) {
|
|
if ($loader instanceof ExistsLoaderInterface && !$loader->exists($name)) {
|
|
continue;
|
|
}
|
|
|
|
try {
|
|
return $loader->getCacheKey($name);
|
|
} catch (LoaderError $e) {
|
|
$exceptions[] = \get_class($loader).': '.$e->getMessage();
|
|
}
|
|
}
|
|
|
|
throw new LoaderError(sprintf('Template "%s" is not defined%s.', $name, $exceptions ? ' ('.implode(', ', $exceptions).')' : ''));
|
|
}
|
|
|
|
public function isFresh($name, $time)
|
|
{
|
|
$exceptions = [];
|
|
foreach ($this->loaders as $loader) {
|
|
if ($loader instanceof ExistsLoaderInterface && !$loader->exists($name)) {
|
|
continue;
|
|
}
|
|
|
|
try {
|
|
return $loader->isFresh($name, $time);
|
|
} catch (LoaderError $e) {
|
|
$exceptions[] = \get_class($loader).': '.$e->getMessage();
|
|
}
|
|
}
|
|
|
|
throw new LoaderError(sprintf('Template "%s" is not defined%s.', $name, $exceptions ? ' ('.implode(', ', $exceptions).')' : ''));
|
|
}
|
|
}
|
|
|
|
class_alias('Twig\Loader\ChainLoader', 'Twig_Loader_Chain');
|