mirror of
https://github.com/slawkens/myaac.git
synced 2025-07-03 02:00:14 +02:00
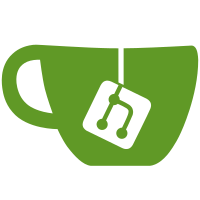
* New admin panel Pages: Options + Config [WIP] * Forgot the plugin example of options * Rename to settings.php * Add Settings Class * New myaac_settings table * Add $limit parameter to $db->select method * Add $member var annotation * Remove useless title_separator from config * Move $menus to menus.php Also fix active link when menu item has subpage * Settings [WIP] New Settings class New Plugins::load() method Move config.php to settings.php MyAAC Settings will have plugin_name = 'core' Add compat_config.php * Change options.php to settings.php * Change name to settings * Add Settings menu * Add Sections + Add setting($key) function Reorganisation * Add email + password fields as type * Update 33.php * add settings migration * php 8 compatibility * add missing hook * Add categories in tabs, move more settings, revert back getPluginSettings Categories and sections are now not numbered Remove example settings plugin * fix typo * Update .gitignore * Add 36th migration for settings table * Execute migrations just after db connect * Update plugins.php * [WIP] Some work on settings Add hidden settings New method: parse, to parse settings from array Move base html to twig template Remove vocation loading from .xml, instead use predefined voc names * Rename * Fix path * [WIP] More work on settings Move more config to settings (mainly mail_* + some other) Remove mail_admin, wasnt used anywhere Add return type to some functions Add Twig settings(key) function Possibility to save setting to db * Add min, max, step to number field option * Re-enable plugin if disabled and already installed * Add Settings menu, including all plugins with settings One change included in previous commit, due to missclick * Nothing important * Better boolean detection * More detailed error message in settings * Lets call it settings.name instead * Add new function: only_if, to hide fields when they are not enabled [WIP] Not fully finished yet * guild_management: show_if * Hide section title on show_if * Fix: check on page load if radio button is checked * Add: show_if - account_mail_verify * nothing important * Rename team_* variables + add to deprecated * Change variable name * Extract Settings:save function * Add settings.callbacks.get * Move forum config to settings * Move status config to settings * Remove whitespaces * More config to settings: account_types, genders, highscores, admin * Move signature config to settings * Move news config to settings * Rename variable * Save config.php in Settings Egg and hen problem solved :) * Test database connection on save settings -> prevents from making website unusable if connection is wrong * Test server_path -> same There is no config.php anymore, just config.local.php, which can be edited manually and also from admin panel * Remove configs from previous commit * Fix create account, if account_create_character_create is enabled * Add more deprecated configs * Add more info into comment * Update 5-database.php * Fix menu highlighting & opening * Update template.php * Enable script option * Reword email settings + move two new settings * add last_kills_limit + move shop * google_analytics_id * add mail_lost_account_interval * Create character blocked words (by @gpedro), just moved to settings * Fix google_analytics * create character name config moved to settings * Fix for install warning - min/max length * New create character checks configurable: block monsters & spells names * fixes * Improve character npc name check * New setting: donate_column + move donate config to settings * Add super fancy No Refresh saving with a toast * Add new possibility: to deny saving setting if condition is not met * Move database settings to separate category * Fix default value displaying * Add database_hash setting * add last_kills_limit to compat config * Move create character blocked names down * Every setting needs to have default * Move rest of config to settings Remove config.php completely Add new settings category: Game Fix account_login_by_email Min textarea size = 2 + adjusted automatically
221 lines
5.9 KiB
PHP
221 lines
5.9 KiB
PHP
<?php
|
|
|
|
use Twig\Environment as Twig_Environment;
|
|
use Twig\Loader\FilesystemLoader as Twig_FilesystemLoader;
|
|
|
|
require '../common.php';
|
|
|
|
define('MYAAC_INSTALL', true);
|
|
|
|
// includes
|
|
require SYSTEM . 'functions.php';
|
|
require BASE . 'install/includes/functions.php';
|
|
require BASE . 'install/includes/locale.php';
|
|
require SYSTEM . 'clients.conf.php';
|
|
require LIBS . 'settings.php';
|
|
|
|
// ignore undefined index from Twig autoloader
|
|
$config['env'] = 'prod';
|
|
|
|
$twig_loader = new Twig_FilesystemLoader(SYSTEM . 'templates');
|
|
$twig = new Twig_Environment($twig_loader, array(
|
|
'cache' => CACHE . 'twig/',
|
|
'auto_reload' => true
|
|
));
|
|
|
|
// load installation status
|
|
$step = $_REQUEST['step'] ?? 'welcome';
|
|
|
|
$install_status = array();
|
|
if(file_exists(CACHE . 'install.txt')) {
|
|
$install_status = unserialize(file_get_contents(CACHE . 'install.txt'));
|
|
|
|
if(!isset($_REQUEST['step'])) {
|
|
$step = isset($install_status['step']) ? $install_status['step'] : '';
|
|
}
|
|
}
|
|
|
|
if(isset($_POST['vars']))
|
|
{
|
|
foreach($_POST['vars'] as $key => $value) {
|
|
$_SESSION['var_' . $key] = $value;
|
|
$install_status[$key] = $value;
|
|
}
|
|
}
|
|
else {
|
|
foreach($install_status as $key => $value) {
|
|
$_SESSION['var_' . $key] = $value;
|
|
}
|
|
}
|
|
|
|
if($step == 'finish' && (!isset($config['installed']) || !$config['installed'])) {
|
|
$step = 'welcome';
|
|
}
|
|
|
|
// step verify
|
|
$steps = array(1 => 'welcome', 2 => 'license', 3 => 'requirements', 4 => 'config', 5 => 'database', 6 => 'admin', 7 => 'finish');
|
|
if(!in_array($step, $steps)) // check if step is valid
|
|
throw new RuntimeException('ERROR: Unknown step.');
|
|
|
|
$install_status['step'] = $step;
|
|
$errors = array();
|
|
|
|
if($step == 'database') {
|
|
foreach($_SESSION as $key => $value) {
|
|
if(strpos($key, 'var_') === false) {
|
|
continue;
|
|
}
|
|
|
|
$key = str_replace('var_', '', $key);
|
|
|
|
if(in_array($key, array('account', 'account_id', 'password', 'password_confirm', 'email', 'player_name'))) {
|
|
continue;
|
|
}
|
|
|
|
if($key != 'usage' && empty($value)) {
|
|
$errors[] = $locale['please_fill_all'];
|
|
break;
|
|
}
|
|
else if($key == 'server_path') {
|
|
$config['server_path'] = $value;
|
|
|
|
// take care of trailing slash at the end
|
|
if($config['server_path'][strlen($config['server_path']) - 1] != '/') {
|
|
$config['server_path'] .= '/';
|
|
}
|
|
|
|
if(!file_exists($config['server_path'] . 'config.lua')) {
|
|
$errors[] = $locale['step_database_error_config'];
|
|
break;
|
|
}
|
|
}
|
|
else if($key == 'timezone' && !in_array($value, DateTimeZone::listIdentifiers())) {
|
|
$errors[] = $locale['step_config_timezone_error'];
|
|
break;
|
|
}
|
|
else if($key == 'client' && !in_array($value, $config['clients'])) {
|
|
$errors[] = $locale['step_config_client_error'];
|
|
break;
|
|
}
|
|
}
|
|
|
|
if(!empty($errors)) {
|
|
$step = 'config';
|
|
}
|
|
}
|
|
else if($step == 'admin') {
|
|
if(!file_exists(BASE . 'config.local.php') || !isset($config['installed']) || !$config['installed']) {
|
|
$step = 'database';
|
|
}
|
|
else {
|
|
$_SESSION['saved'] = true;
|
|
}
|
|
}
|
|
else if($step == 'finish') {
|
|
$email = $_SESSION['var_email'];
|
|
$password = $_SESSION['var_password'];
|
|
$password_confirm = $_SESSION['var_password_confirm'];
|
|
$player_name = $_SESSION['var_player_name'];
|
|
|
|
// email check
|
|
if(empty($email)) {
|
|
$errors[] = $locale['step_admin_email_error_empty'];
|
|
}
|
|
else if(!Validator::email($email)) {
|
|
$errors[] = $locale['step_admin_email_error_format'];
|
|
}
|
|
|
|
// account check
|
|
if(isset($_SESSION['var_account'])) {
|
|
if(empty($_SESSION['var_account'])) {
|
|
$errors[] = $locale['step_admin_account_error_empty'];
|
|
}
|
|
else if(!Validator::accountName($_SESSION['var_account'])) {
|
|
$errors[] = $locale['step_admin_account_error_format'];
|
|
}
|
|
else if(strtoupper($_SESSION['var_account']) == strtoupper($password)) {
|
|
$errors[] = $locale['step_admin_account_error_same'];
|
|
}
|
|
}
|
|
else if(isset($_SESSION['var_account_id'])) {
|
|
if(empty($_SESSION['var_account_id'])) {
|
|
$errors[] = $locale['step_admin_account_id_error_empty'];
|
|
}
|
|
else if(!Validator::accountId($_SESSION['var_account_id'])) {
|
|
$errors[] = $locale['step_admin_account_id_error_format'];
|
|
}
|
|
else if($_SESSION['var_account_id'] == $password) {
|
|
$errors[] = $locale['step_admin_account_id_error_same'];
|
|
}
|
|
}
|
|
|
|
// password check
|
|
if(empty($password)) {
|
|
$errors[] = $locale['step_admin_password_error_empty'];
|
|
}
|
|
else if(!Validator::password($password)) {
|
|
$errors[] = $locale['step_admin_password_error_format'];
|
|
}
|
|
else if($password != $password_confirm) {
|
|
$errors[] = $locale['step_admin_password_confirm_error_not_same'];
|
|
}
|
|
|
|
// player name check
|
|
if(empty($player_name)) {
|
|
$errors[] = $locale['step_admin_player_name_error_empty'];
|
|
}
|
|
else if(!Validator::characterName($player_name)) {
|
|
$errors[] = $locale['step_admin_player_name_error_format'];
|
|
}
|
|
|
|
if(!empty($errors)) {
|
|
$step = 'admin';
|
|
}
|
|
}
|
|
|
|
if(empty($errors)) {
|
|
file_put_contents(CACHE . 'install.txt', serialize($install_status));
|
|
}
|
|
|
|
$error = false;
|
|
|
|
clearstatcache();
|
|
if(is_writable(CACHE) && (MYAAC_OS != 'WINDOWS' || win_is_writable(CACHE))) {
|
|
if(!file_exists(BASE . 'install/ip.txt')) {
|
|
$content = warning('AAC installation is disabled. To enable it make file <b>ip.txt</b> in install/ directory and put there your IP.<br/>
|
|
Your IP is:<br /><b>' . $_SERVER['REMOTE_ADDR'] . '</b>', true);
|
|
}
|
|
else {
|
|
$file_content = trim(file_get_contents(BASE . 'install/ip.txt'));
|
|
$allow = false;
|
|
$listIP = preg_split('/\s+/', $file_content);
|
|
foreach($listIP as $ip) {
|
|
if($_SERVER['REMOTE_ADDR'] == $ip) {
|
|
$allow = true;
|
|
}
|
|
}
|
|
|
|
if(!$allow)
|
|
{
|
|
$content = warning('In file <b>install/ip.txt</b> must be your IP!<br/>
|
|
In file is:<br /><b>' . nl2br($file_content) . '</b><br/>
|
|
Your IP is:<br /><b>' . $_SERVER['REMOTE_ADDR'] . '</b>', true);
|
|
}
|
|
else {
|
|
ob_start();
|
|
|
|
$step_id = array_search($step, $steps);
|
|
require 'steps/' . $step_id . '-' . $step . '.php';
|
|
$content = ob_get_contents();
|
|
ob_end_clean();
|
|
}
|
|
}
|
|
}
|
|
else {
|
|
$content = error(file_get_contents(BASE . 'install/includes/twig_error.html'), true);
|
|
}
|
|
|
|
// render
|
|
require 'template/template.php';
|
|
//$_SESSION['laststep'] = $step;
|