mirror of
https://github.com/slawkens/myaac.git
synced 2025-07-03 02:00:14 +02:00
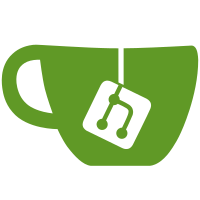
* (internal) new function: OTS_Guild::hasMember(OTS_Player $player) * (internal) new function: Forum::hasAccess($board_id)
114 lines
5.7 KiB
PHP
114 lines
5.7 KiB
PHP
<?php
|
|
/**
|
|
* New forum post
|
|
*
|
|
* @package MyAAC
|
|
* @author Gesior <jerzyskalski@wp.pl>
|
|
* @author Slawkens <slawkens@gmail.com>
|
|
* @copyright 2017 MyAAC
|
|
* @version 0.6.1
|
|
* @link http://my-aac.org
|
|
*/
|
|
defined('MYAAC') or die('Direct access not allowed!');
|
|
|
|
if(Forum::canPost($account_logged))
|
|
{
|
|
$players_from_account = $db->query("SELECT `players`.`name`, `players`.`id` FROM `players` WHERE `players`.`account_id` = ".(int) $account_logged->getId())->fetchAll();
|
|
$thread_id = isset($_REQUEST['thread_id']) ? (int) $_REQUEST['thread_id'] : 0;
|
|
if($thread_id == 0) {
|
|
echo "Thread with this id doesn't exist.";
|
|
return;
|
|
}
|
|
|
|
$thread = $db->query("SELECT `" . TABLE_PREFIX . "forum`.`post_topic`, `" . TABLE_PREFIX . "forum`.`id`, `" . TABLE_PREFIX . "forum`.`section` FROM `" . TABLE_PREFIX . "forum` WHERE `" . TABLE_PREFIX . "forum`.`id` = ".(int) $thread_id." AND `" . TABLE_PREFIX . "forum`.`first_post` = ".(int) $thread_id." LIMIT 1")->fetch();
|
|
echo '<a href="' . getLink('forum') . '">Boards</a> >> <a href="' . getForumBoardLink($thread['section']) . '">'.$sections[$thread['section']]['name'].'</a> >> <a href="' . getForumThreadLink($thread_id) . '">'.$thread['post_topic'].'</a> >> <b>Post new reply</b><br /><h3>'.$thread['post_topic'].'</h3>';
|
|
if(isset($thread['id']) && Forum::hasAccess($thread['section']))
|
|
{
|
|
$quote = isset($_REQUEST['quote']) ? (int) $_REQUEST['quote'] : NULL;
|
|
$text = isset($_REQUEST['text']) ? stripslashes(trim($_REQUEST['text'])) : NULL;
|
|
$char_id = (int) (isset($_REQUEST['char_id']) ? $_REQUEST['char_id'] : 0);
|
|
$post_topic = isset($_REQUEST['topic']) ? stripslashes(trim($_REQUEST['topic'])) : '';
|
|
$smile = (int) (isset($_REQUEST['smile']) ? $_REQUEST['smile'] : 0);
|
|
$saved = false;
|
|
if(isset($_REQUEST['quote']))
|
|
{
|
|
$quoted_post = $db->query("SELECT `players`.`name`, `" . TABLE_PREFIX . "forum`.`post_text`, `" . TABLE_PREFIX . "forum`.`post_date` FROM `players`, `" . TABLE_PREFIX . "forum` WHERE `players`.`id` = `" . TABLE_PREFIX . "forum`.`author_guid` AND `" . TABLE_PREFIX . "forum`.`id` = ".(int) $quote)->fetchAll();
|
|
if(isset($quoted_post[0]['name']))
|
|
$text = '[i]Originally posted by '.$quoted_post[0]['name'].' on '.date('d.m.y H:i:s', $quoted_post[0]['post_date']).':[/i][quote]'.$quoted_post[0]['post_text'].'[/quote]';
|
|
}
|
|
elseif(isset($_REQUEST['save']))
|
|
{
|
|
$lenght = 0;
|
|
for($i = 0; $i < strlen($text); $i++)
|
|
{
|
|
if(ord($text[$i]) >= 33 && ord($text[$i]) <= 126)
|
|
$lenght++;
|
|
}
|
|
if($lenght < 1 || strlen($text) > 15000)
|
|
$errors[] = 'Too short or too long post (short: '.$lenght.' long: '.strlen($text).' letters). Minimum 1 letter, maximum 15000 letters.';
|
|
if($char_id == 0)
|
|
$errors[] = 'Please select a character.';
|
|
|
|
$player_on_account = false;
|
|
if(count($errors) == 0)
|
|
{
|
|
foreach($players_from_account as $player)
|
|
if($char_id == $player['id'])
|
|
$player_on_account = true;
|
|
if(!$player_on_account)
|
|
$errors[] = 'Player with selected ID '.$char_id.' doesn\'t exist or isn\'t on your account';
|
|
}
|
|
if(count($errors) == 0)
|
|
{
|
|
$last_post = 0;
|
|
$query = $db->query('SELECT post_date FROM ' . TABLE_PREFIX . 'forum ORDER BY post_date DESC LIMIT 1');
|
|
if($query->rowCount() > 0)
|
|
{
|
|
$query = $query->fetch();
|
|
$last_post = $query['post_date'];
|
|
}
|
|
if($last_post+$config['forum_post_interval']-time() > 0 && !Forum::isModerator())
|
|
$errors[] = 'You can post one time per '.$config['forum_post_interval'].' seconds. Next post after '.($last_post+$config['forum_post_interval']-time()).' second(s).';
|
|
}
|
|
if(count($errors) == 0)
|
|
{
|
|
$saved = true;
|
|
Forum::add_post($thread['id'], $thread['section'], $account_logged->getId(), (int) $char_id, $text, $post_topic, (int) $smile, time(), $_SERVER['REMOTE_ADDR']);
|
|
$db->query("UPDATE `" . TABLE_PREFIX . "forum` SET `replies`=`replies`+1, `last_post`=".time()." WHERE `id` = ".(int) $thread_id);
|
|
$post_page = $db->query("SELECT COUNT(`" . TABLE_PREFIX . "forum`.`id`) AS posts_count FROM `players`, `" . TABLE_PREFIX . "forum` WHERE `players`.`id` = `" . TABLE_PREFIX . "forum`.`author_guid` AND `" . TABLE_PREFIX . "forum`.`post_date` <= ".time()." AND `" . TABLE_PREFIX . "forum`.`first_post` = ".(int) $thread['id'])->fetch();
|
|
$_page = (int) ceil($post_page['posts_count'] / $config['forum_threads_per_page']) - 1;
|
|
header('Location: ' . getForumThreadLink($thread_id, $_page));
|
|
echo '<br />Thank you for posting.<br /><a href="' . getForumThreadLink($thread_id, $_page) . '">GO BACK TO LAST THREAD</a>';
|
|
}
|
|
}
|
|
|
|
if(!$saved)
|
|
{
|
|
if(!empty($errors))
|
|
echo $twig->render('error_box.html.twig', array('errors' => $errors));
|
|
|
|
$threads = $db->query("SELECT `players`.`name`, `" . TABLE_PREFIX . "forum`.`post_text`, `" . TABLE_PREFIX . "forum`.`post_topic`, `" . TABLE_PREFIX . "forum`.`post_smile` FROM `players`, `" . TABLE_PREFIX . "forum` WHERE `players`.`id` = `" . TABLE_PREFIX . "forum`.`author_guid` AND `" . TABLE_PREFIX . "forum`.`first_post` = ".(int) $thread_id." ORDER BY `" . TABLE_PREFIX . "forum`.`post_date` DESC LIMIT 5")->fetchAll();
|
|
|
|
foreach($threads as &$thread) {
|
|
$thread['post'] = Forum::showPost($thread['post_topic'], $thread['post_text'], $thread['post_smile']);
|
|
}
|
|
|
|
echo $twig->render('forum.new_post.html.twig', array(
|
|
'thread_id' => $thread_id,
|
|
'post_player_id' => $char_id,
|
|
'players' => $players_from_account,
|
|
'post_topic' => $post_topic,
|
|
'post_text' => $text,
|
|
'post_smile' => $smile,
|
|
'topic' => $thread['post_topic'],
|
|
'threads' => $threads
|
|
));
|
|
}
|
|
}
|
|
else
|
|
echo "Thread with ID " . $thread_id . " doesn't exist.";
|
|
}
|
|
else
|
|
echo "Your account is banned, deleted or you don't have any player with level " . $config['forum_level_required'] . " on your account. You can't post.";
|
|
|
|
?>
|