mirror of
https://github.com/slawkens/myaac.git
synced 2025-07-02 01:30:14 +02:00
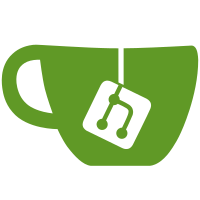
This fixes some errors on PHP 7.4 and contains even more fixes Also bumped PHP version to 5.5 as Twig requires it.
102 lines
2.6 KiB
PHP
102 lines
2.6 KiB
PHP
<?php
|
|
|
|
/*
|
|
* This file is part of Twig.
|
|
*
|
|
* (c) Fabien Potencier
|
|
*
|
|
* For the full copyright and license information, please view the LICENSE
|
|
* file that was distributed with this source code.
|
|
*/
|
|
|
|
namespace Twig\Extension;
|
|
|
|
use Twig\Environment;
|
|
use Twig\NodeVisitor\NodeVisitorInterface;
|
|
use Twig\TokenParser\TokenParserInterface;
|
|
use Twig\TwigFilter;
|
|
use Twig\TwigFunction;
|
|
use Twig\TwigTest;
|
|
|
|
/**
|
|
* Interface implemented by extension classes.
|
|
*
|
|
* @author Fabien Potencier <fabien@symfony.com>
|
|
*/
|
|
interface ExtensionInterface
|
|
{
|
|
/**
|
|
* Initializes the runtime environment.
|
|
*
|
|
* This is where you can load some file that contains filter functions for instance.
|
|
*
|
|
* @deprecated since 1.23 (to be removed in 2.0), implement \Twig_Extension_InitRuntimeInterface instead
|
|
*/
|
|
public function initRuntime(Environment $environment);
|
|
|
|
/**
|
|
* Returns the token parser instances to add to the existing list.
|
|
*
|
|
* @return TokenParserInterface[]
|
|
*/
|
|
public function getTokenParsers();
|
|
|
|
/**
|
|
* Returns the node visitor instances to add to the existing list.
|
|
*
|
|
* @return NodeVisitorInterface[]
|
|
*/
|
|
public function getNodeVisitors();
|
|
|
|
/**
|
|
* Returns a list of filters to add to the existing list.
|
|
*
|
|
* @return TwigFilter[]
|
|
*/
|
|
public function getFilters();
|
|
|
|
/**
|
|
* Returns a list of tests to add to the existing list.
|
|
*
|
|
* @return TwigTest[]
|
|
*/
|
|
public function getTests();
|
|
|
|
/**
|
|
* Returns a list of functions to add to the existing list.
|
|
*
|
|
* @return TwigFunction[]
|
|
*/
|
|
public function getFunctions();
|
|
|
|
/**
|
|
* Returns a list of operators to add to the existing list.
|
|
*
|
|
* @return array<array> First array of unary operators, second array of binary operators
|
|
*/
|
|
public function getOperators();
|
|
|
|
/**
|
|
* Returns a list of global variables to add to the existing list.
|
|
*
|
|
* @return array An array of global variables
|
|
*
|
|
* @deprecated since 1.23 (to be removed in 2.0), implement \Twig_Extension_GlobalsInterface instead
|
|
*/
|
|
public function getGlobals();
|
|
|
|
/**
|
|
* Returns the name of the extension.
|
|
*
|
|
* @return string The extension name
|
|
*
|
|
* @deprecated since 1.26 (to be removed in 2.0), not used anymore internally
|
|
*/
|
|
public function getName();
|
|
}
|
|
|
|
class_alias('Twig\Extension\ExtensionInterface', 'Twig_ExtensionInterface');
|
|
|
|
// Ensure that the aliased name is loaded to keep BC for classes implementing the typehint with the old aliased name.
|
|
class_exists('Twig\Environment');
|