mirror of
https://github.com/slawkens/myaac.git
synced 2025-07-03 02:00:14 +02:00
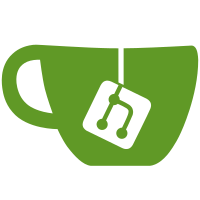
* wip * wip * wip * wip * wip * fix: reusing pdo connection from pot * wip * wip * wip * wip * move files In future, all classes will be in src/ folder * Replace namespace name, for future * Remove duplicated exception * Fix towns from db * Fix spells page * Add default FAQ question + FAQ model * feat: reset colors in menus * Add confirm + save button at the top (menus) * Do not insert duplicated FAQ on install * Refactor install menus * Fix changelogs showing * Fix menu update, only with specified template name * Fix account create -> missing compat * Fix bans_per_page * banned_by is player_id. type = 2 is namelock in tfs 0.3 * Add getPlayerNameById, fix getPlayerNameByAccount * Change link name * Order by lastlogin * fix: query optimize * wip * wip * wip * wip * wip * wip * wip * Refactor notepad.php, class was useless * This is showing error, if the updated rows = 0 * Fix success & error class (bootstrap) * Uncomment require migrate.php * Some distro have owner_id * Update Player.php --------- Co-authored-by: slawkens <slawkens@gmail.com>
147 lines
2.7 KiB
PHP
147 lines
2.7 KiB
PHP
<?php
|
|
|
|
use MyAAC\Models\Changelog as ModelsChangelog;
|
|
|
|
class Changelog
|
|
{
|
|
static public function verify($body,$date, &$errors)
|
|
{
|
|
if(!isset($date) || !isset($body[0])) {
|
|
$errors[] = 'Please fill all inputs.';
|
|
return false;
|
|
}
|
|
|
|
if(strlen($body) > CL_LIMIT) {
|
|
$errors[] = 'Changelog content cannot be longer than ' . CL_LIMIT . ' characters.';
|
|
return false;
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
static public function add($body, $type, $where, $player_id, $cdate, &$errors)
|
|
{
|
|
if(!self::verify($body,$cdate, $errors))
|
|
return false;
|
|
|
|
$row = new ModelsChangelog;
|
|
$row->body = $body;
|
|
$row->type = $type;
|
|
$row->date = $cdate;
|
|
$row->where = $where;
|
|
$row->player_id = $player_id ?? 0;
|
|
if ($row->save()) {
|
|
self::clearCache();
|
|
return true;
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
static public function get($id) {
|
|
return ModelsChangelog::find($id);
|
|
}
|
|
|
|
static public function update($id, $body, $type, $where, $player_id, $date, &$errors)
|
|
{
|
|
if(!self::verify($body,$date, $errors))
|
|
return false;
|
|
|
|
if (ModelsChangelog::where('id', '=', $id)->update([
|
|
'body' => $body,
|
|
'type' => $type,
|
|
'where' => $where,
|
|
'player_id' => $player_id ?? 0,
|
|
'date' => $date
|
|
])) {
|
|
self::clearCache();
|
|
return true;
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
static public function delete($id, &$errors)
|
|
{
|
|
if(isset($id))
|
|
{
|
|
$row = ModelsChangelog::find($id);
|
|
if ($row) {
|
|
if (!$row->delete()) {
|
|
$errors[] = 'Fail during delete Changelog.';
|
|
}
|
|
} else {
|
|
$errors[] = 'Changelog with id ' . $id . ' does not exist.';
|
|
}
|
|
} else {
|
|
$errors[] = 'Changelog id not set.';
|
|
}
|
|
|
|
if(count($errors)) {
|
|
return false;
|
|
}
|
|
|
|
self::clearCache();
|
|
return true;
|
|
}
|
|
|
|
static public function toggleHidden($id, &$errors, &$status)
|
|
{
|
|
if(isset($id))
|
|
{
|
|
$row = ModelsChangelog::find($id);
|
|
if ($row) {
|
|
$row->hidden = $row->hidden == 1 ? 0 : 1;
|
|
if (!$row->save()) {
|
|
$errors[] = 'Fail during toggle hidden Changelog.';
|
|
}
|
|
} else {
|
|
$errors[] = 'Changelog with id ' . $id . ' does not exists.';
|
|
}
|
|
|
|
}
|
|
else
|
|
$errors[] = 'Changelog id not set.';
|
|
|
|
if(count($errors)) {
|
|
return false;
|
|
}
|
|
|
|
self::clearCache();
|
|
return true;
|
|
}
|
|
|
|
static public function getCached($type)
|
|
{
|
|
global $template_name;
|
|
|
|
$cache = Cache::getInstance();
|
|
if ($cache->enabled())
|
|
{
|
|
$tmp = '';
|
|
if ($cache->fetch('changelog_' . $template_name, $tmp) && isset($tmp[0])) {
|
|
return $tmp;
|
|
}
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
static public function clearCache()
|
|
{
|
|
global $template_name;
|
|
$cache = Cache::getInstance();
|
|
if (!$cache->enabled()) {
|
|
return;
|
|
}
|
|
|
|
$tmp = '';
|
|
foreach (get_templates() as $template) {
|
|
if ($cache->fetch('changelog_' . $template_name, $tmp)) {
|
|
$cache->delete('changelog_' . $template_name);
|
|
}
|
|
|
|
}
|
|
}
|
|
}
|