mirror of
https://github.com/slawkens/myaac.git
synced 2025-07-06 03:30:15 +02:00
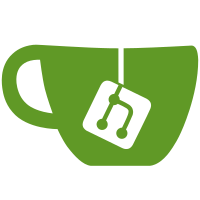
* Migrations up down * Add forum model * Syntactic sugar for db structure changes * Refactor migrations with $up & $down * Fix migrations upgrade and downgrade + Add option to disable auto migrate * Add migrate:to command Usage: php aac migrate:to x (x - database version) * Show error when mail is not enabled * Fixes regarding to init.php * Add migrate command to manually upgrade db, incase auto migrate is disabled * Fixed rest of the migrations * Limit max version of database * Don't allow minus number * Option to clear specified plugin settings by name * Version is required * Fix PHPStan errors * Unset $up after migration, to prevent executing same migration twice * Add database version to output * This is not needed * Update 5.php * Set database_auto_migrate on install * Set blank & color only if current db version supports it * Fix duplicate function declaration
53 lines
1.4 KiB
PHP
53 lines
1.4 KiB
PHP
<?php
|
|
|
|
namespace MyAAC\Commands;
|
|
|
|
use MyAAC\Plugins;
|
|
use Symfony\Component\Console\Input\InputArgument;
|
|
use Symfony\Component\Console\Input\InputInterface;
|
|
use Symfony\Component\Console\Output\OutputInterface;
|
|
use Symfony\Component\Console\Style\SymfonyStyle;
|
|
|
|
class PluginInstallCommand extends Command
|
|
{
|
|
protected function configure(): void
|
|
{
|
|
$this->setName('plugin:install')
|
|
->setDescription('This command installs plugin')
|
|
->addArgument('pathToPluginZip', InputArgument::REQUIRED, 'Path to zip file (plugin) that you want to install');
|
|
}
|
|
|
|
protected function execute(InputInterface $input, OutputInterface $output): int
|
|
{
|
|
require SYSTEM . 'init.php';
|
|
|
|
$io = new SymfonyStyle($input, $output);
|
|
|
|
$pathToFile = $input->getArgument('pathToPluginZip');
|
|
|
|
$ext = strtolower(pathinfo($pathToFile, PATHINFO_EXTENSION));
|
|
if($ext !== 'zip') {// check if it is zipped/compressed file
|
|
$io->error('Please install only .zip files');
|
|
return 2;
|
|
}
|
|
|
|
if(!file_exists($pathToFile)) {
|
|
$io->error('File ' . $pathToFile . ' does not exist');
|
|
return 3;
|
|
}
|
|
|
|
if(!Plugins::install($pathToFile)){
|
|
$io->error(Plugins::getError());
|
|
return 4;
|
|
}
|
|
|
|
foreach(Plugins::getWarnings() as $warning) {
|
|
$io->warning($warning);
|
|
}
|
|
|
|
$info = Plugins::getPluginJson();
|
|
$io->success((isset($info['name']) ? $info['name'] . ' p' : 'P') . 'lugin has been successfully installed.');
|
|
return Command::SUCCESS;
|
|
}
|
|
}
|