mirror of
https://github.com/slawkens/myaac.git
synced 2025-04-26 01:09:21 +02:00
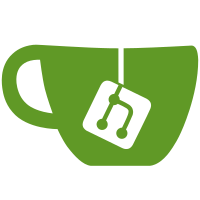
* Migrations up down * Add forum model * Syntactic sugar for db structure changes * Refactor migrations with $up & $down * Fix migrations upgrade and downgrade + Add option to disable auto migrate * Add migrate:to command Usage: php aac migrate:to x (x - database version) * Show error when mail is not enabled * Fixes regarding to init.php * Add migrate command to manually upgrade db, incase auto migrate is disabled * Fixed rest of the migrations * Limit max version of database * Don't allow minus number * Option to clear specified plugin settings by name * Version is required * Fix PHPStan errors * Unset $up after migration, to prevent executing same migration twice * Add database version to output * This is not needed * Update 5.php * Set database_auto_migrate on install * Set blank & color only if current db version supports it * Fix duplicate function declaration
65 lines
1.5 KiB
PHP
65 lines
1.5 KiB
PHP
<?php
|
|
|
|
namespace MyAAC\Commands;
|
|
|
|
use Symfony\Component\Console\Input\InputArgument;
|
|
use Symfony\Component\Console\Input\InputInterface;
|
|
use Symfony\Component\Console\Output\OutputInterface;
|
|
use Symfony\Component\Console\Style\SymfonyStyle;
|
|
|
|
class MigrateRunCommand extends Command
|
|
{
|
|
protected function configure(): void
|
|
{
|
|
$this->setName('migrate:run')
|
|
->setDescription('This command runs the migration specified by id')
|
|
->addArgument('id',
|
|
InputArgument::IS_ARRAY | InputArgument::REQUIRED,
|
|
'Id or ids of migration(s)'
|
|
);
|
|
}
|
|
|
|
protected function execute(InputInterface $input, OutputInterface $output): int
|
|
{
|
|
require SYSTEM . 'init.php';
|
|
|
|
$io = new SymfonyStyle($input, $output);
|
|
|
|
$ids = $input->getArgument('id');
|
|
|
|
// pre-check
|
|
// in case one of the migrations doesn't exist - we won't execute any of them
|
|
foreach ($ids as $id) {
|
|
if (!$this->migrationExists($id)) {
|
|
$io->error([
|
|
"One of the migrations specified doesnt exist: $id",
|
|
"Please check it and re-run the command",
|
|
"No migration has been executed",
|
|
]);
|
|
|
|
return Command::FAILURE;
|
|
}
|
|
}
|
|
|
|
foreach ($ids as $id) {
|
|
$this->executeMigration($id, $io);
|
|
}
|
|
|
|
return Command::SUCCESS;
|
|
}
|
|
|
|
private function migrationExists($id): bool {
|
|
return file_exists(SYSTEM . 'migrations/' . $id . '.php');
|
|
}
|
|
|
|
private function executeMigration($id, $io): void
|
|
{
|
|
global $db;
|
|
|
|
$db->revalidateCache();
|
|
|
|
require SYSTEM . 'migrations/' . $id . '.php';
|
|
$io->success('Migration ' . $id . ' successfully executed');
|
|
}
|
|
}
|